How to Build Real-Time Dashboards Using Splunk and React
Date Published
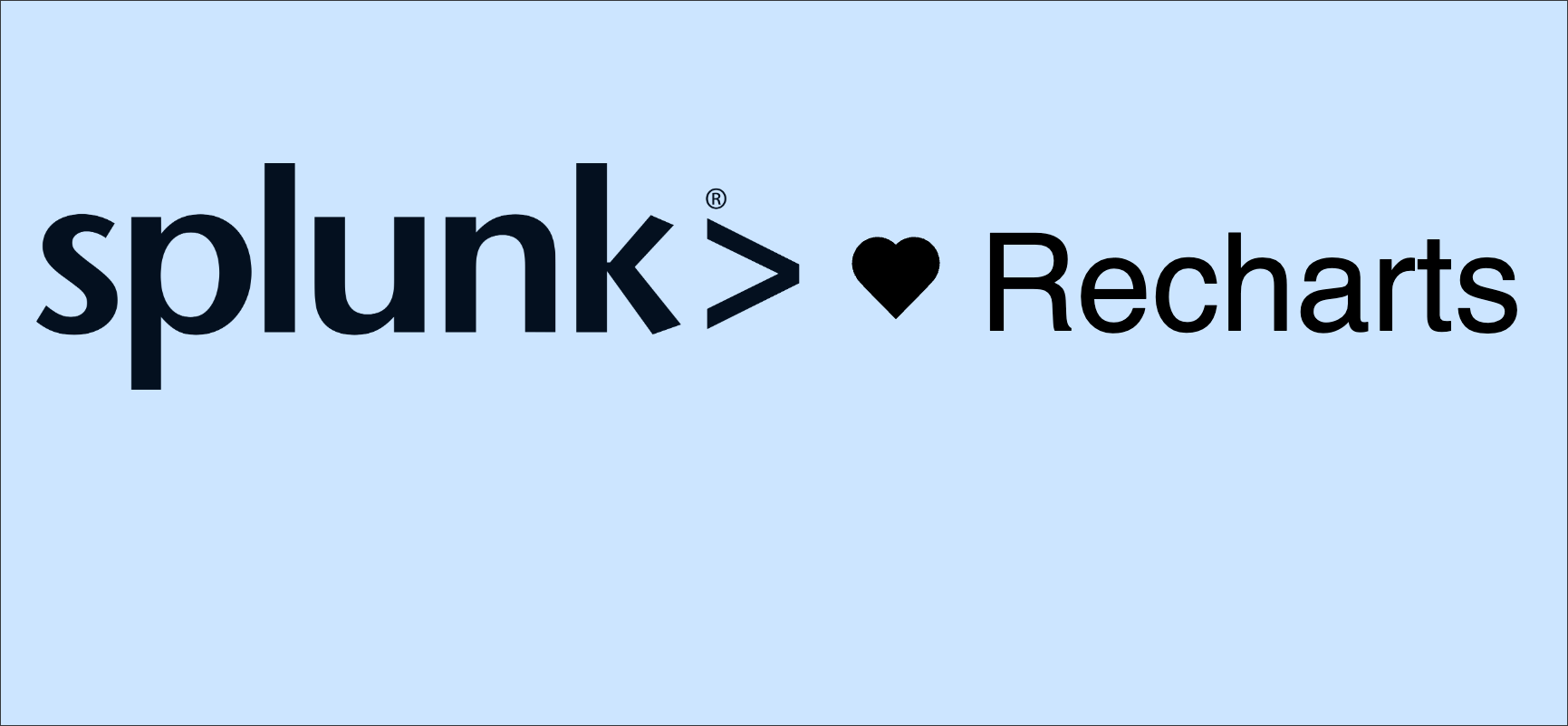
How to Build Real-Time Dashboards Using Splunk and React
Real-time dashboards provide immediate insights into data, enabling faster decision-making. Combining Splunk—a powerful data analysis and visualization platform—with React, a popular JavaScript library for building user interfaces, you can create interactive and dynamic dashboards that update in real time. In this guide, we’ll walk through the steps to integrate Splunk with React to build an efficient real-time dashboard.
Why Use Splunk and React Together?
Splunk excels at indexing and analyzing large datasets, making it a robust backend for data insights. React’s component-based structure enables the creation of intuitive, dynamic, and reusable user interfaces. When combined, they form a powerful duo for building dashboards that provide real-time updates and detailed analytics.
Prerequisites
Splunk: Ensure you have access to a Splunk instance with the required datasets indexed.
React: Basic knowledge of React and a project scaffolded using Create React App or Vite.
Splunk Python SDK: Used for querying Splunk data programmatically.
WebSocket Server: For real-time updates.
Backend Service: A custom service to interface with Splunk and stream data to the frontend.
Step 1: Setting Up the Backend with Splunk Python SDK
The backend will act as a bridge between Splunk and the React app, fetching data from Splunk and broadcasting it via WebSocket for real-time updates.
Install Splunk Python SDK:
1pip install splunk-sdk
Authenticate with Splunk: Use the SDK to connect to your Splunk instance:
1import splunklib.client as client23splunk_service = client.connect(4 host="<splunk-host>",5 port=8089,6 username="<username>",7 password="<password>"8)
Fetch Data: Query Splunk to fetch data. For example, retrieve log entries:
Set Up WebSocket: Use a WebSocket library like websockets to stream data:
1import asyncio2import websockets34async def send_updates(websocket, path):5 while True:6 data = fetch_logs()7 await websocket.send(json.dumps(data))8 await asyncio.sleep(5)910asyncio.get_event_loop().run_until_complete(11 websockets.serve(send_updates, "localhost", 8765)12)13asyncio.get_event_loop().run_forever()
Step 2: Setting Up the React Frontend
Install Dependencies: Add libraries for WebSocket communication and charting:
1npm install recharts
Create WebSocket Connection: Establish a WebSocket connection in your React app:
1import React, { useEffect, useState } from 'react';23const useWebSocket = (url: string) => {4 const [data, setData] = useState([]);56 useEffect(() => {7 const ws = new WebSocket(url);8 ws.onmessage = (event) => {9 setData(JSON.parse(event.data));10 };11 return () => ws.close();12 }, [url]);1314 return data;15};1617export default useWebSocket;
Visualize Data: Use Recharts to display data:
1import { LineChart, Line, CartesianGrid, XAxis, YAxis, Tooltip } from 'recharts';23const Dashboard = () => {4 const data = useWebSocket('ws://localhost:8765');56 return (7 <div>8 <h1>Real-Time Dashboard</h1>9 <LineChart width={600} height={300} data={data}>10 <Line type="monotone" dataKey="count" stroke="#8884d8" />11 <CartesianGrid stroke="#ccc" />12 <XAxis dataKey="source" />13 <YAxis />14 <Tooltip />15 </LineChart>16 </div>17 );18};1920export default Dashboard;21
Step 3: Deploying the Dashboard
Backend Deployment:
Use a cloud provider like AWS or Azure to host your backend service.
Ensure Splunk is accessible from the deployed backend.
Frontend Deployment:
Build the React app using:
1npm run build
Deploy using a platform like Netlify, Vercel, or your web server.
Connect Frontend to Backend: Update the WebSocket URL in the frontend to point to the backend’s WebSocket server.
Final Thoughts
By combining the analytical power of Splunk with the interactivity of React, you can build real-time dashboards tailored to your needs. This setup is scalable and flexible, allowing you to enhance your dashboard with features like filters, authentication, and advanced visualizations. Experiment with this approach to unlock the full potential of your data!
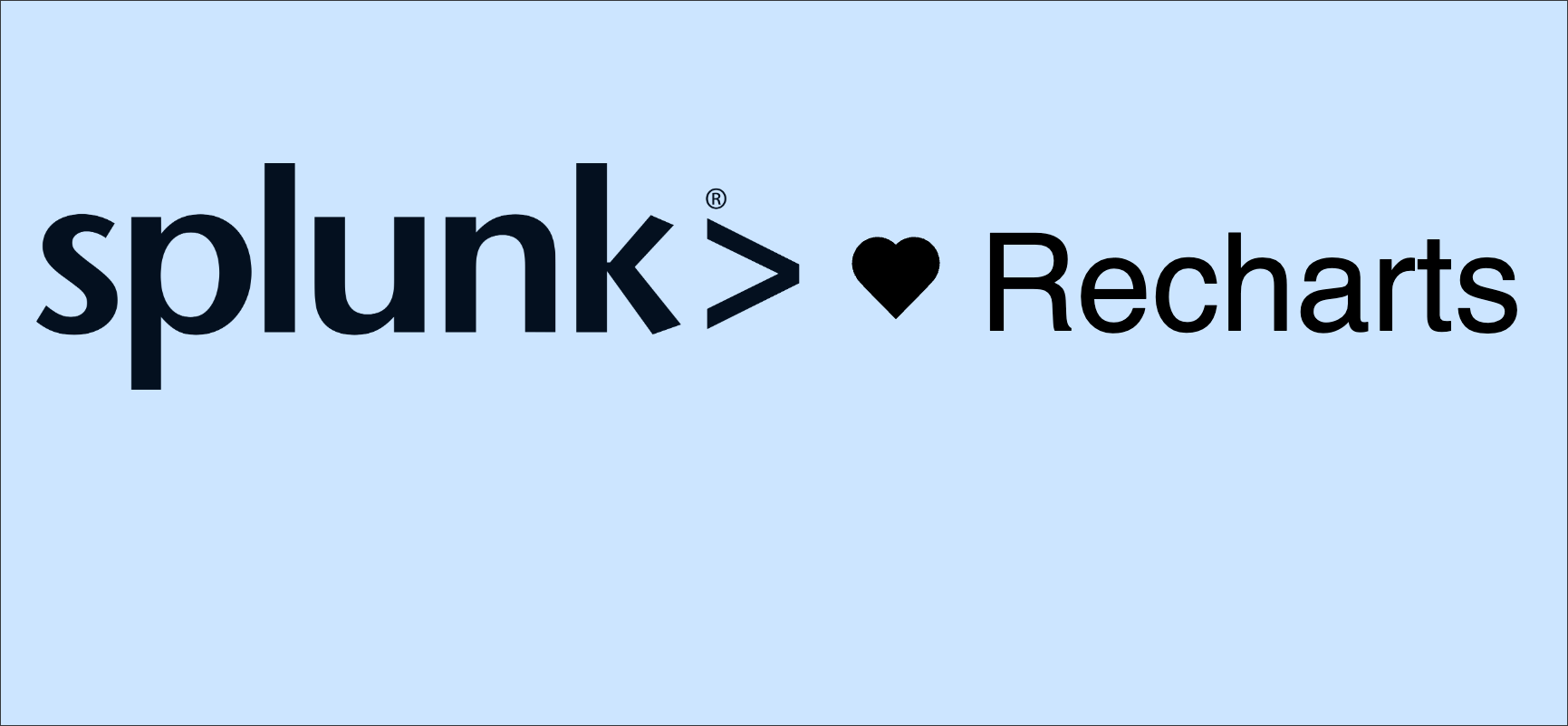
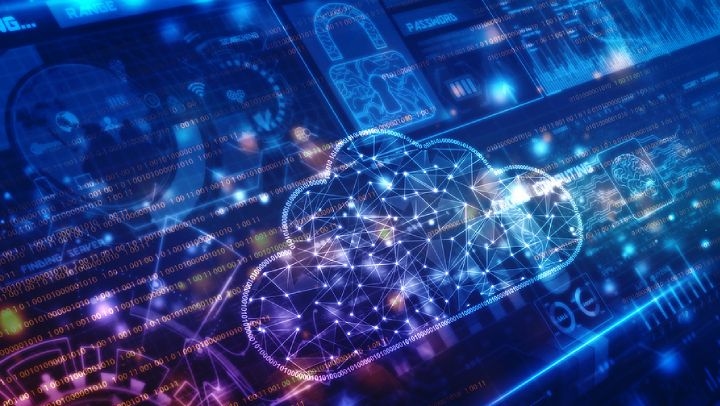
In an increasingly interconnected digital world, businesses often need to share resources, communication channels securely with other organizations.